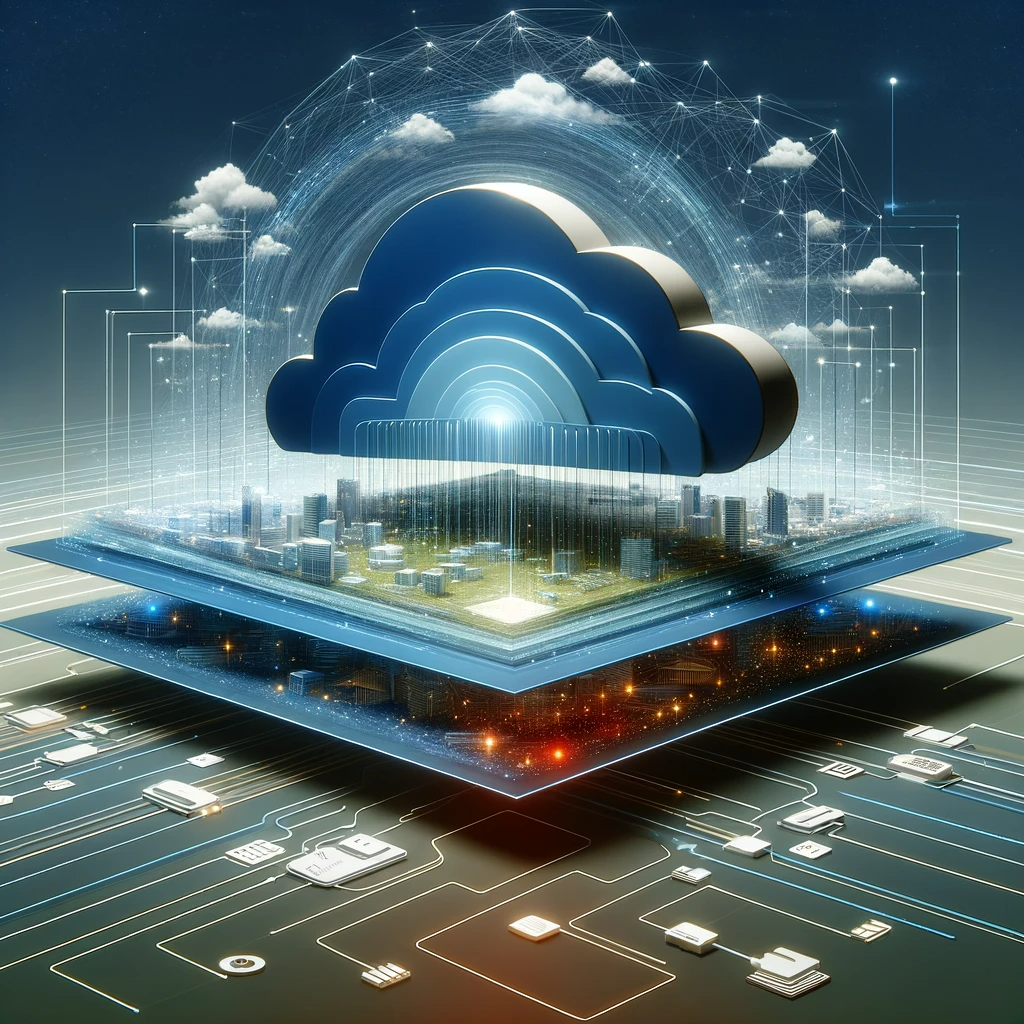
Imagine the journey of “Global Shopper,” an e-commerce platform that began as a modest local online store and gradually transformed into a sprawling international marketplace. This growth trajectory presented a critical challenge — the need to integrate multiple payment gateways to cater to a diverse global audience. As Global Shopper expanded, it encountered varied payment preferences, currencies, and customer expectations across different regions. The complexity of managing this variety in payment methods and currencies demanded a robust and adaptable solution. This led to the development of a Django-based payment abstraction layer, further refined with a dynamic payment strategy model, proving to be an invaluable asset in the platform’s global expansion.
The Necessity of a Payment Abstraction Layer
A payment abstraction layer serves as a crucial intermediary between an application and multiple payment gateways. Its primary role is to streamline the integration of new payment options, significantly simplifying codebase maintenance. This layer is especially crucial for businesses experiencing rapid global expansion, allowing them to swiftly adapt to varying market needs and preferences.
Expanding Use Cases
Consider the following scenarios where a payment abstraction layer becomes essential:
- Regional Payment Preferences: In Europe, platforms might prioritize integrating with PayPal or SEPA (Single Euro Payments Area) for direct bank transfers, while in Asia, services like Alipay or WeChat Pay are more prevalent. A payment abstraction layer allows e-commerce platforms to seamlessly switch or add these services without overhauling their existing systems.
- Currency Handling: Dealing with multiple currencies is another challenge. An advanced payment abstraction layer can facilitate currency conversions and handle transactions in local currencies, enhancing customer experience.
- Compliance and Security: Different regions have varying compliance requirements (like GDPR in Europe or PCI DSS for card payments). A well-structured payment layer can help in aligning with these standards while maintaining high security for transactions.
- Subscription Models: For businesses with subscription-based services, managing recurring payments across different gateways becomes simpler with a unified abstraction layer.
- Refund and Cancellation Handling: Managing refunds and cancellations efficiently across different gateways is another capability that can be streamlined through a payment abstraction layer.
Why Django?
While the concept of a payment abstraction layer is language-agnostic and can be implemented in various programming environments, Django, a high-level Python web framework, stands out for its comprehensive approach. Known for its “batteries-included” philosophy, Django offers an extensive range of tools and features right out of the box. Its ORM (Object-Relational Mapping) system, class-based views, and MVC (Model-View-Controller) architecture make it particularly suited for developing complex systems like a payment abstraction layer.
The Versatility of Programming Languages
It’s important to note that the principles of building a payment abstraction layer are not confined to Django or Python. They can be translated and applied in other programming environments as well.
- JavaScript with Node.js: For applications running on a JavaScript stack, Node.js can be used to create a similar backend solution, leveraging its vast npm ecosystem.
- Ruby on Rails: Known for its convention over configuration philosophy, Ruby on Rails can also be an efficient choice for such implementations, especially for rapid application development.
- Java with Spring Framework: For enterprise-level applications, Java with Spring Framework offers robustness and scalability, which can be essential for handling complex payment processing requirements.
- PHP with Laravel: PHP, with frameworks like Laravel, can also be used to build a payment abstraction layer, especially in scenarios where existing e-commerce platforms are built with PHP.
By understanding the requirements of a payment abstraction layer and applying the appropriate design patterns, developers can implement this system in the language and framework that best fits their project’s needs.
Building the Foundation: Setting Up Django
Here we will use Django as an example to build such a system. before diving into the payment system, ensure you have Django installed and a project set up:
pip install django
django-admin startproject payment_project
cd payment_project
python manage.py startapp payment
This creates a new Django project and a dedicated app for handling payment functionalities.
Step 1: Designing the Payment Interface
The payment interface is the core of the abstraction layer. It defines common methods that all payment gateways must implement. Create a PaymentGateway
interface in payment/interfaces.py
:
class PaymentGateway:
def process_payment(self, amount, currency):
raise NotImplementedError
def refund_payment(self, transaction_id):
raise NotImplementedError
This interface includes methods for processing payments and issuing refunds, standard operations for most payment gateways.
Step 2: Implementing Gateway-Specific Classes
For each payment gateway (like Stripe, PayPal, etc.), you’ll create a class implementing the PaymentGateway
interface. This is where you'll integrate the specific API logic of each payment gateway.
In payment/gateways.py
, define classes for different gateways:
from .interfaces import PaymentGateway
class StripePaymentGateway(PaymentGateway):
def process_payment(self, amount, currency):
# Integration logic with Stripe API
pass
def refund_payment(self, transaction_id):
# Stripe refund logic
pass
class PayPalPaymentGateway(PaymentGateway):
def process_payment(self, amount, currency):
# Integration logic with PayPal API
pass
def refund_payment(self, transaction_id):
# PayPal refund logic
pass
These classes encapsulate the specific API calls and business logic for each payment provider.
Step 3: Creating a Payment Strategy Model
The payment strategy model is a Django model that dictates which payment gateway to use based on transaction data like amount and currency. This model allows GlobalShopper to dynamically select the most appropriate payment gateway.
Define the model in payment/models.py
:
from django.db import models
class PaymentStrategy(models.Model):
gateway_name = models.CharField(max_length=100)
preferred_currency = models.CharField(max_length=3) # ISO currency code
min_amount = models.DecimalField(max_digits=10, decimal_places=2)
def __str__(self):
return f"{self.gateway_name} - {self.preferred_currency} - {self.min_amount}"
Here, gateway_name
represents the payment gateway, preferred_currency
indicates the currency code (like USD, EUR), and min_amount
is the minimum transaction amount for which this gateway is preferred.
Step 4: Utilizing the Strategy in Views
In Django views, use the strategy model to select the appropriate payment gateway based on the transaction details. In payment/views.py
:
from django.http import JsonResponse
from .models import PaymentStrategy
from .gateways import StripePaymentGateway, PayPalPaymentGateway
def process_payment(request, amount, currency):
strategy = PaymentStrategy.objects.filter(preferred_currency=currency, min_amount__lte=amount).first()
if not strategy:
return JsonResponse({'error': 'No suitable payment strategy found'}, status=400)
# Dynamic gateway selection based on the strategy
if strategy.gateway_name == 'stripe':
gateway = StripePaymentGateway()
elif strategy.gateway_name == 'paypal':
gateway = PayPalPaymentGateway()
# ... other gateways
response = gateway.process_payment(amount, currency)
return JsonResponse(response)
This view function dynamically selects a payment gateway based on the currency and amount of the transaction, using the strategy defined in the database.
Conclusion: Embracing a Global Payment Strategy
Implementing a payment abstraction layer with a dynamic payment strategy model in Django allows GlobalShopper to efficiently handle a variety of payment methods and currencies, crucial for its international clientele. This system not only streamlines payment processing but also enhances the user experience by accommodating their preferred payment methods.
Encouraging Experimentation and Feedback
The journey doesn’t end here. Experiment with different gateways, try out various strategies, and adapt the model to fit your specific business needs. Share your experiences, challenges, and successes in the comments. Your insights could help others embarking on a similar journey.
"interface" - Google News
January 28, 2024 at 01:02AM
https://ift.tt/Xzl6uD7
Streamlining E-commerce: Navigating Payment Interfaces with Abstraction Layers — Insights and… - Medium
"interface" - Google News
https://ift.tt/mJauhqv
https://ift.tt/dlA6F7w
Bagikan Berita Ini
0 Response to "Streamlining E-commerce: Navigating Payment Interfaces with Abstraction Layers — Insights and… - Medium"
Post a Comment